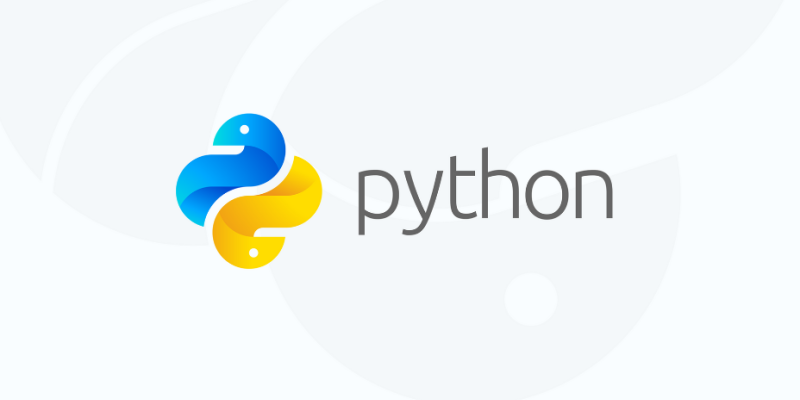
What is a syntax?
In computer programming, syntax defines the rules that guide the structure and manner in which a program is written.
These rules are important because they allow a compiler or an interpreter such as the Python interpreter to understand the the program or language to be executed by the computer.
Compilers simply convert a program into a language that the computer understands. This language is called a binary code. While programming languages such as C, C#, C++, Java, and more use compilers, Python does not, but rather uses an interpreter.
An interpreter simply executes a program at the runtime. In both cases, if the syntax is wrong or doesn’t follow the defined rules for the language, the code execution will fail as well as return errors.
Indentation
When we write a program, we typically have a problem we want to solve. This often involves a few steps that could represent a function that helps us to address a problem or execute a task. This piece of code can be seen as a block. Very quickly, we can have a program with many blocks of code before we can fully solve a problem or complete a task.
In Python, indentations are used to specify what block of code is related and to differentiate a block from others.
Let’s consider a simple Python program that helps us to perform two tasks namely; addition and multiplication of any two numbers provided as an input.
We have written two functions add_two_numbers()
and multiply_two_numbers()
.
These functions require two numbers as input to carry out the computation.
We have used indentations to specify the line of codes that below to the specific functions or code block.
Indentations can be done with a single tab or four whitespaces as seen below.
def add_two_numbers(a,b):
c = a+b
print(c)
def multiply_two_numbers(x,y):
z = x*y
print(z)
add_two_numbers(5,7)
multiply_two_numbers(2,2)
Output:
12
4
A few things to note;
You cannot indent the first line of code.
If you decide to use 4 whitespaces or a single tab space, stick with it through out your program.
Indentation does not only allow the interpreter to understand your code, but it also provides a structure that makes it easy to read and understand.
Identifiers
In simple terms, an identifier is a name that helps us differentiate something from others in a code.
This could be a name used to identify a variable, function, class, module, and more.
A few rules to remember
Identifies can include a combination of alphabets, numbers, or underscore.
Some words are reserved in Python and cannot be used as identifiers.
Symbols and special characters are not acceptable.
Identifiers cannot begin with a digit.
Identifiers are case sensitive meaning that “myvar” is different from “MYVAR”.
Examples are shown below;
firstName = "Johnny"
myAddress1 ="10 Alton Avenue"
my_age=18
var=100
MYVAR=99
var2=45
Python also allows you to check if the name chosen is a valid and acceptable name for an identifier. This can be done using an in-built function called “isidentifier()”.
Example as shown below;
print("myVar123".isidentifier())
Output:
True
print("123var".isidentifier())
Output:
False
Keywords
As mentioned earlier, Python has some words reserved and cannot be used as identifiers.
To view a list of these words, use the following command shown below;
import keyword
print(keyword.kwlist)
Output:
[‘False’, ‘None’, ‘True’, ‘__peg_parser__’, ‘and’, ‘as’, ‘assert’, ‘async’, ‘await’, ‘break’, ‘class’, ‘continue’, ‘def’, ‘del’, ‘elif’, ‘else’, ‘except’, ‘finally’, ‘for’, ‘from’, ‘global’, ‘if’, ‘import’, ‘in’, ‘is’, ‘lambda’, ‘nonlocal’, ‘not’, ‘or’, ‘pass’, ‘raise’, ‘return’, ‘try’, ‘while’, ‘with’, ‘yield’]
Comments
To write a comment within your Python program, we simply use the symbol (#) before the comment. This is referred to as a single-line comment.
Comments tell the computer which lines within a program are not to be executed (i.e. ignored) at runtime.
Example
#This is a comment. print("I am a comment")
print("Hello")
Output:
Hello
Writing multiple lines of comments in Python is also possible. For this, we use three double quotations as shown in the example below.
"""
This is a comment.
print("I am a comment")
"""
print("Hello")
Output:
Hello
Statements
A statement is a line of code such as the example shown below. In Python, we can have multiple statements in one line as well.
a = 10 #this is a statment.
b = 5; c = 6; d = 7 #these are multiple statements written in one line.